目次
オブジェクト指向 Python 継承
Pyhonによるオブジェクト指向プログラミングのサンプルプログラムです
継承の目的
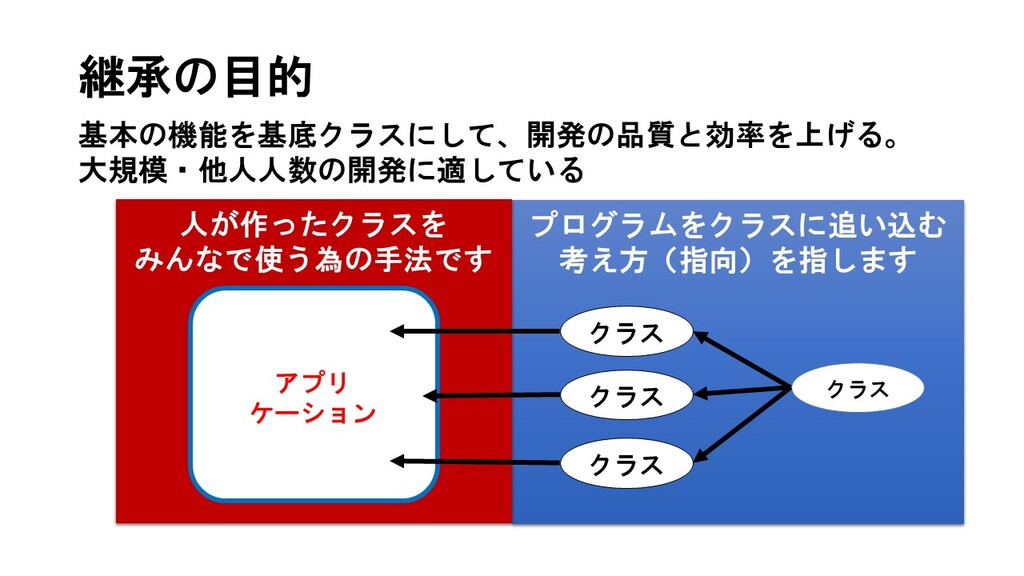
継承とインスタンス化(inheritance)
基底クラスを継承してクラスを作ると両方のメソッドが使える
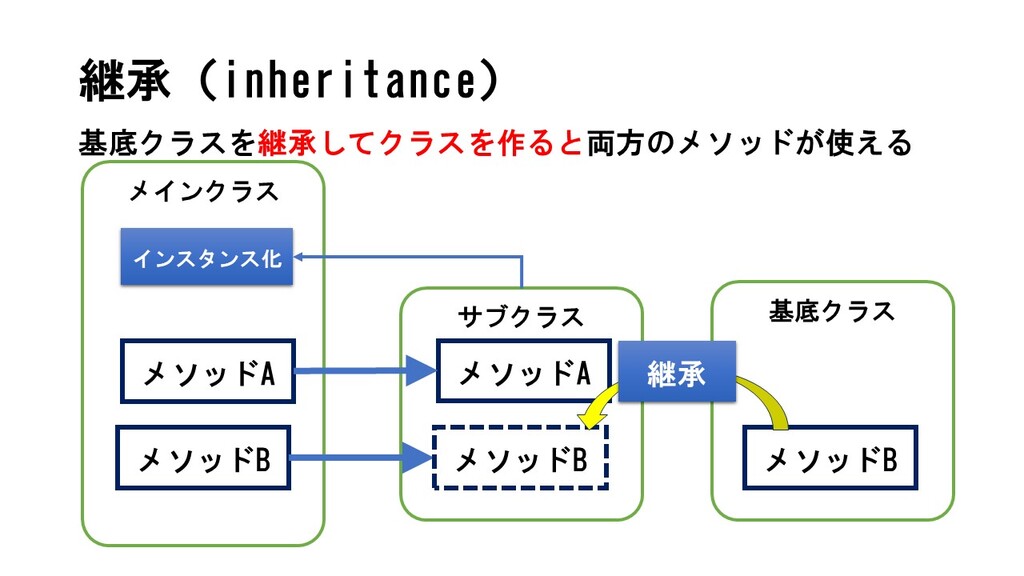
事例
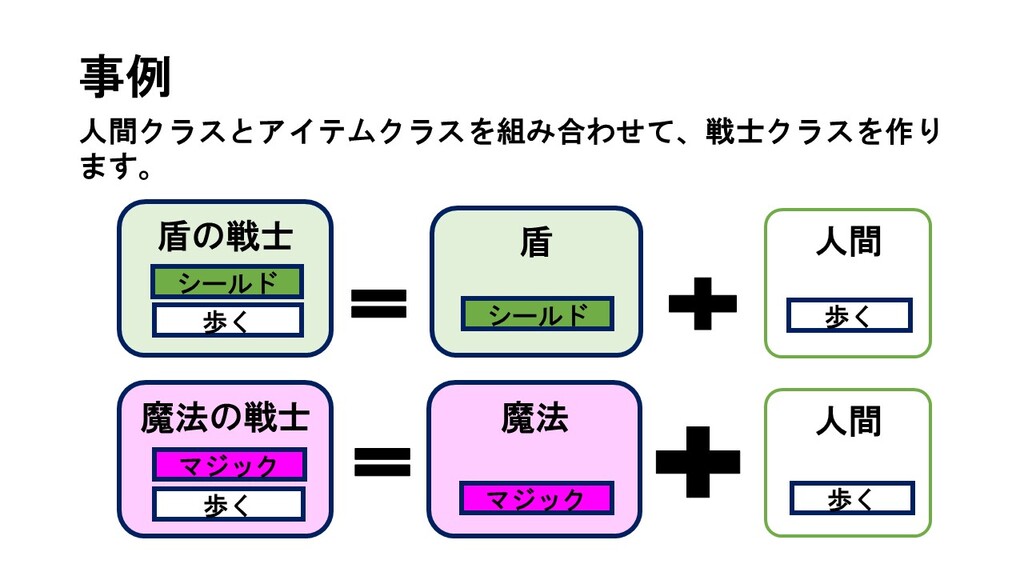
継承
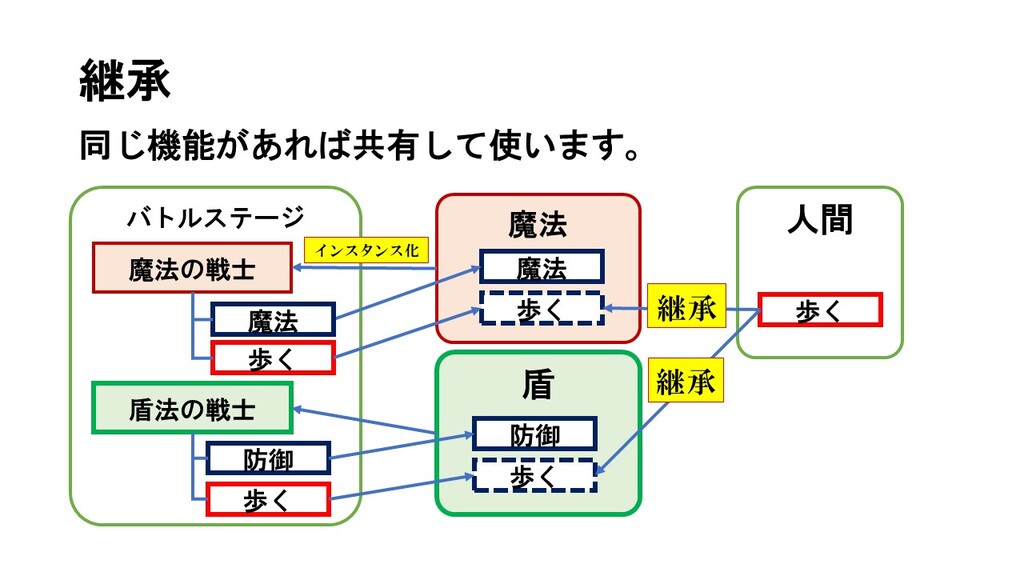
オーバーライド(上書き)
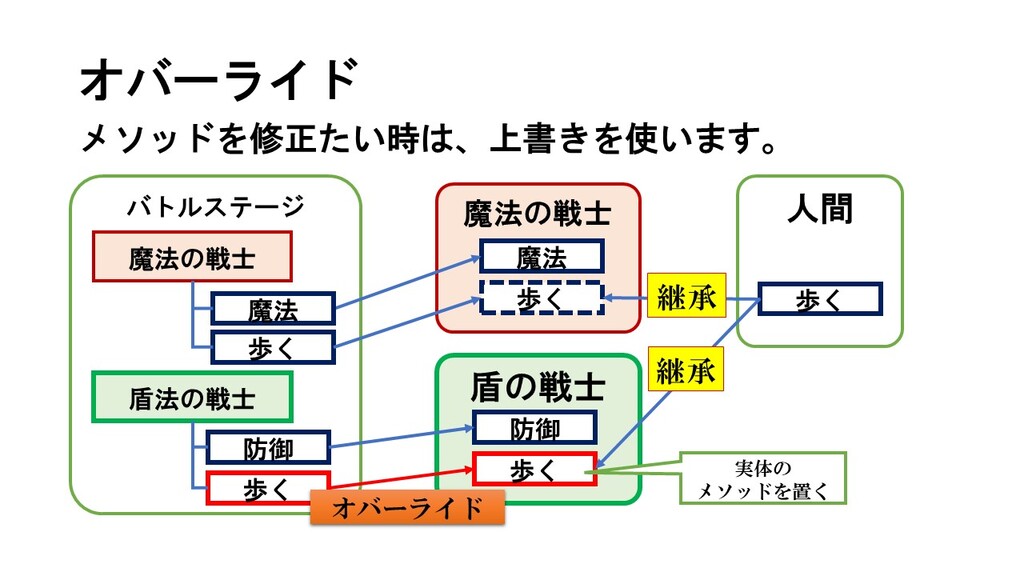
ソースコード
カラー表示用のクラス
参照
野村数学研究所
あわせて読みたい
基底クラス
人間のクラスを作成します。
class Human(): name = "Human" attribute = "Human" cWalk = 20 ; sWalk = "." tColor = Color.WHITE ; rColor = Color.RESET def __init__(self, n ): self.name = n def Walk(self): s = "" for i in range(self.cWalk): s += self.sWalk print(self.attribute,self.tColor,s,"(",self.name,")",self.rColor) def Run(self): s = "" for i in range(self.cWalk): s += self.sWalk+self.sWalk print(self.attribute,self.tColor,s,"(",self.name,")",self.rColor)
盾の戦士 クラス
基底クラスを継承した。サブクラスを作成します。
class Shielder(Human): name = "Shielder" attribute = "Shielder" tColor = Color.RED ; rColor = Color.RESET cshield = 5 ; sshield = "@" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor )
魔法の戦士 クラス (人間クラスを継承)
class Wizard(Human): name = "Wizard" attribute = "Wizard" tColor = Color.GREEN ; rColor = Color.RESET cshield = 20 ; sshield = "~" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor )
メインクラス
class subMain: # humanList = ["Nao*","Itsu*","Moto*","Re*"] # hu = dict() s = Shielder( "Itsu*" ) w = Wizard( "Moto*" ) s.Defense() w.Attack() if __name__ == "__main__": root = subMain()
全ソース
class Color: # 野村数学研究所 : https://www.nomuramath.com/kv8wr0mp/https://www.nomuramath.com/kv8wr0mp/ BLACK = '\033[30m'#(文字)黒 RED = '\033[31m'#(文字)赤 GREEN = '\033[32m'#(文字)緑 YELLOW = '\033[33m'#(文字)黄 BLUE = '\033[34m'#(文字)青 MAGENTA = '\033[35m'#(文字)マゼンタ CYAN = '\033[36m'#(文字)シアン WHITE = '\033[37m'#(文字)白 COLOR_DEFAULT = '\033[39m'#文字色をデフォルトに戻す BOLD = '\033[1m'#太字 UNDERLINE = '\033[4m'#下線 INVISIBLE = '\033[08m'#不可視 REVERCE = '\033[07m'#文字色と背景色を反転 BG_BLACK = '\033[40m'#(背景)黒 BG_RED = '\033[41m'#(背景)赤 BG_GREEN = '\033[42m'#(背景)緑 BG_YELLOW = '\033[43m'#(背景)黄 BG_BLUE = '\033[44m'#(背景)青 BG_MAGENTA = '\033[45m'#(背景)マゼンタ BG_CYAN = '\033[46m'#(背景)シアン BG_WHITE = '\033[47m'#(背景)白 BG_DEFAULT = '\033[49m'#背景色をデフォルトに戻す RESET = '\033[0m'#全てリセット class Human(): name = "Human" attribute = "Human" cWalk = 20 ; sWalk = "." tColor = Color.WHITE ; rColor = Color.RESET def __init__(self, n ): self.name = n def Walk(self): s = "" for i in range(self.cWalk): s += self.sWalk print(self.attribute,self.tColor,s,"(",self.name,")",self.rColor) def Run(self): s = "" for i in range(self.cWalk): s += self.sWalk+self.sWalk print(self.attribute,self.tColor,s,"(",self.name,")",self.rColor) class Shielder(Human): name = "Shielder" attribute = "Shielder" tColor = Color.RED ; rColor = Color.RESET cshield = 5 ; sshield = "@" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) class Wizard(Human): name = "Wizard" attribute = "Wizard" tColor = Color.GREEN ; rColor = Color.RESET cshield = 20 ; sshield = "~" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cshield): s += self.sshield print(self.attribute,self.tColor, s, "(" , self.name, ")", self.rColor ) class subMain: # humanList = ["Nao*","Itsu*","Moto*","Re*"] # hu = dict() s = Shielder( "Itsu*" ) w = Wizard( "Moto*" ) s.Defense() w.Attack() if __name__ == "__main__": root = subMain()