目次
オブジェクト指向 オブジェクト指向
インターフェイスの目的
抽象化により、異なるクラスを同じ様に取り扱いできます。
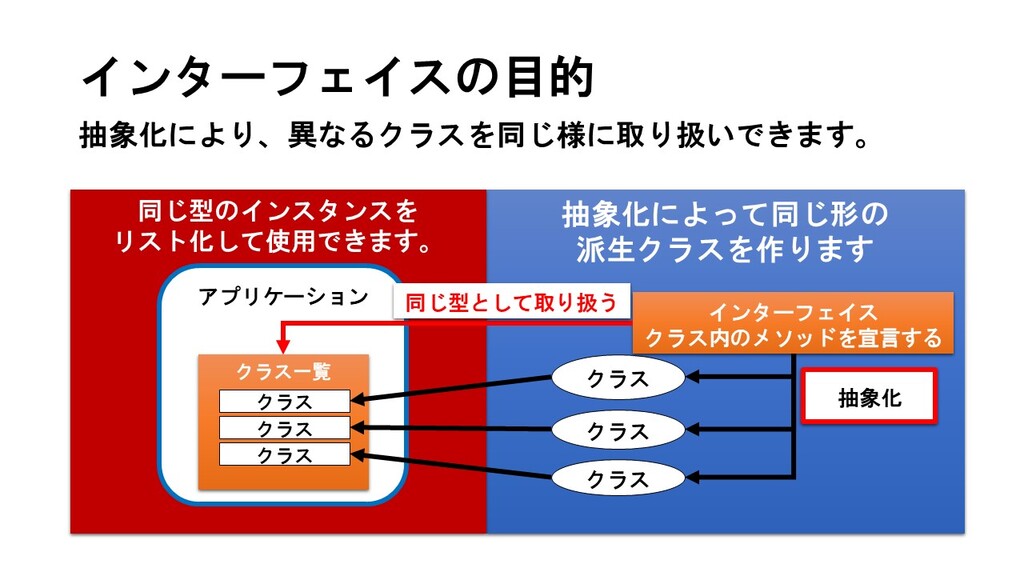
インターフェイスのメリット
作る側 ・クラスの外部仕様が明確になります。 使う側 ・異なるクラスを同じ様に取り扱いできます。 ・クラスのバリエーションを増やす場合に仕様が明確になります。 同じメソッドを作れば良い ・完成したクラスが無くても、メインプログラムが作成できます 仕様(使うメソッド)が判っているので 他のクラスを代用したり、空のテストクラスの作成できます
インターフェイスの作り方
メソッドの抽象化
各クラスのメソッド名を抽象化して統一して、インターフェイスクラスを作ります。
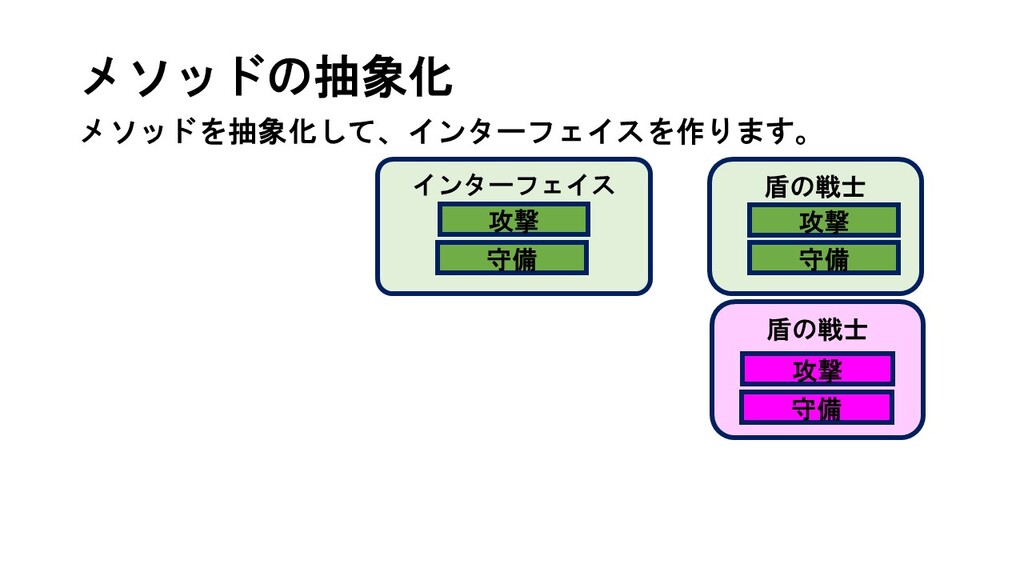
クラスの登録
インターフェイスの基底クラスとして登録
""" インターフエイスの規定クラスとして登録 """ iAdventurer.register(Shielder) iAdventurer.register(Wizard) iAdventurer.register(Swords)
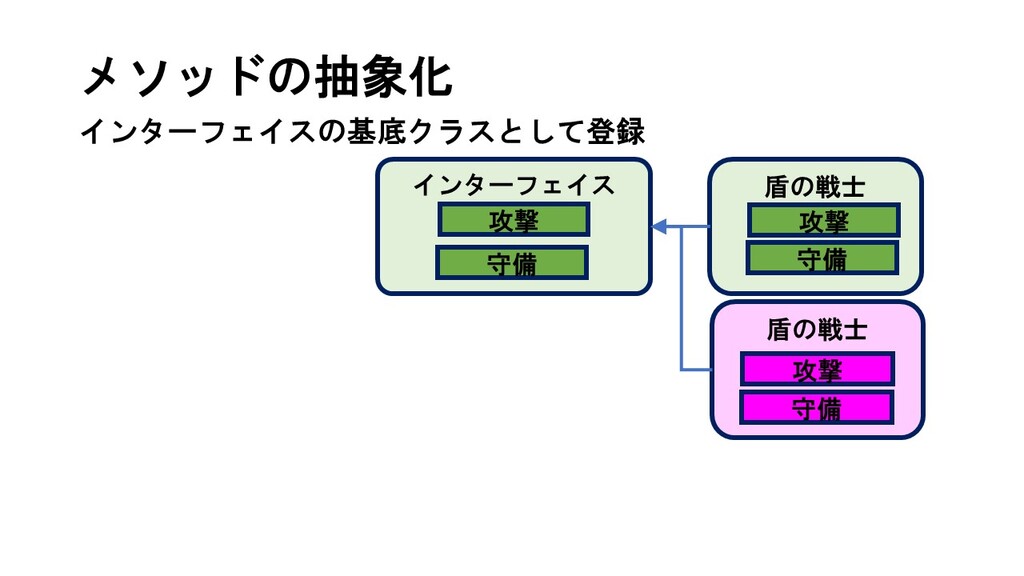
リストの作成
クラスをリストに登録する事で一括して扱えます。
# クラスをリスト化 Adventurers = [Shielder("Nao") , Wizard("Itsu"), Swords("Motoya")]
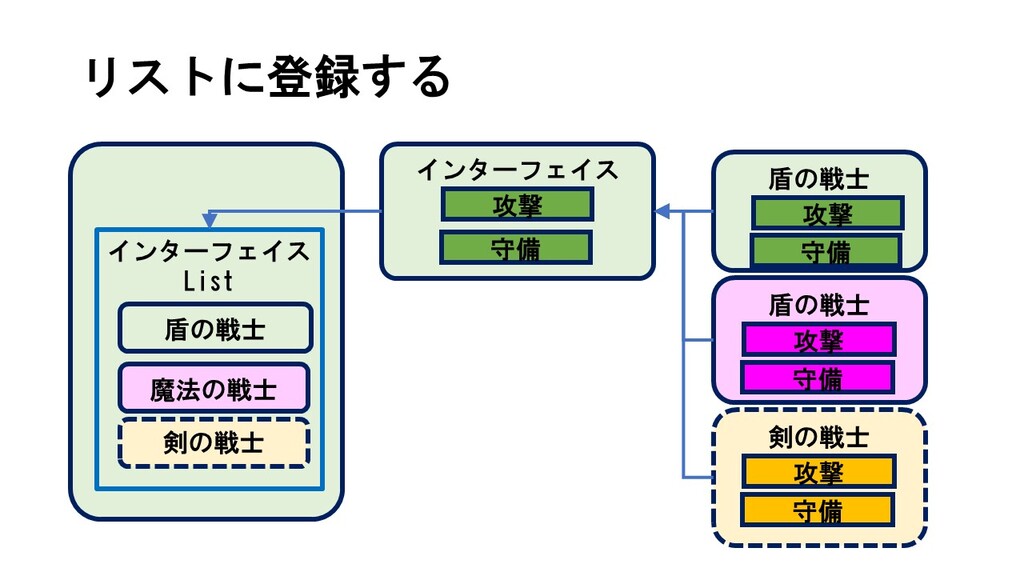
使い方
for Adventurer in Adventurers: if isinstance(Adventurer, iAdventurer): print( f"属性:{Adventurer.attribute} 名前:{Adventurer.name} ") Adventurer.Attack() Adventurer.Defense() Adventurer.Walk()
ソースコード
インターフェイス用ライブラリ
import abc
カラー表示用のクラス
野村数学研究所
あわせて読みたい
class Color: # 野村数学研究所 : https://www.nomuramath.com/kv8wr0mp/https://www.nomuramath.com/kv8wr0mp/ BLACK = '\033[30m'#(文字)黒 RED = '\033[31m'#(文字)赤 GREEN = '\033[32m'#(文字)緑 YELLOW = '\033[33m'#(文字)黄 BLUE = '\033[34m'#(文字)青 MAGENTA = '\033[35m'#(文字)マゼンタ CYAN = '\033[36m'#(文字)シアン WHITE = '\033[37m'#(文字)白 COLOR_DEFAULT = '\033[39m'#文字色をデフォルトに戻す BOLD = '\033[1m'#太字 UNDERLINE = '\033[4m'#下線 INVISIBLE = '\033[08m'#不可視 REVERCE = '\033[07m'#文字色と背景色を反転 BG_BLACK = '\033[40m'#(背景)黒 BG_RED = '\033[41m'#(背景)赤 BG_GREEN = '\033[42m'#(背景)緑 BG_YELLOW = '\033[43m'#(背景)黄 BG_BLUE = '\033[44m'#(背景)青 BG_MAGENTA = '\033[45m'#(背景)マゼンタ BG_CYAN = '\033[46m'#(背景)シアン BG_WHITE = '\033[47m'#(背景)白 BG_DEFAULT = '\033[49m'#背景色をデフォルトに戻す RESET = '\033[0m'#全てリセット
基底クラス
class Human(): """ 基底クラス """ name = "Human" attribute = "Human" tColor = Color.WHITE ; rColor = Color.RESET cWalk = 20; sWalk = "." def __init__(self, n ): self.name = n def Walk(self): s = "" for i in range(self.cWalk): s += self.sWalk print(self.attribute,"\t","w", self.tColor,s,"(",self.name,")",self.rColor)
魔法使いクラス
class Wizard(Human): name = "wizard" attribute = "Wizard" tColor = Color.MAGENTA ; rColor = Color.RESET cAttack = 20 ; sAttack = "@" cDifence = 10 ; sDifence = ":" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cDifence): s += self.sDifence print(self.attribute,"\t","D", self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cAttack): s += self.sAttack print(self.attribute,"\t", "A",self.tColor, s, "(" , self.name, ")", self.rColor )
盾の戦士クラス
class Shielder(Human): name = "Shield" attribute = "Shield" tColor = Color.GREEN ; rColor = Color.RESET cAttack = 20 ; sAttack = "→" cDifence = 10 ; sDifence = "-" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cDifence ): s += self.sDifence print(self.attribute,"\t","D", self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cAttack): s += self.sAttack print(self.attribute,"\t","A",self.tColor, s, "(" , self.name, ")", self.rColor )
剣士クラス(未作成分)
class Swords(Human): name = "Swords" attribute = "Swords" tColor = Color.CYAN ; rColor = Color.RESET cAttack = 20 ; sAttack = "/" cDifence = 10 ; sDifence = ">" def __init__(self, n ): self.name = n def Defense(self): pass def Attack(self): pass
インターフエイス
class iAdventurer(metaclass=abc.ABCMeta): """ インターフェイス """ def Attack(self): pass def Defense(self): pass
メインクラス
class subMain: #humanList = ["Nao*","Itsu*","Motoya","Re*"] """ インターフエイスの規定クラスとして登録 """ iAdventurer.register(Shielder) iAdventurer.register(Wizard) iAdventurer.register(Swords) # クラスをリスト化 Adventurers = [Shielder("Nao") , Wizard("Itsu"), Swords("Motoya")] for Adventurer in Adventurers: if isinstance(Adventurer, iAdventurer): print( f"属性:{Adventurer.attribute} 名前:{Adventurer.name} ") Adventurer.Attack() Adventurer.Defense() Adventurer.Walk() if __name__ == "__main__": root = subMain()
全ソース
import abc class Color: # 野村数学研究所 : https://www.nomuramath.com/kv8wr0mp/https://www.nomuramath.com/kv8wr0mp/ BLACK = '\033[30m'#(文字)黒 RED = '\033[31m'#(文字)赤 GREEN = '\033[32m'#(文字)緑 YELLOW = '\033[33m'#(文字)黄 BLUE = '\033[34m'#(文字)青 MAGENTA = '\033[35m'#(文字)マゼンタ CYAN = '\033[36m'#(文字)シアン WHITE = '\033[37m'#(文字)白 COLOR_DEFAULT = '\033[39m'#文字色をデフォルトに戻す BOLD = '\033[1m'#太字 UNDERLINE = '\033[4m'#下線 INVISIBLE = '\033[08m'#不可視 REVERCE = '\033[07m'#文字色と背景色を反転 BG_BLACK = '\033[40m'#(背景)黒 BG_RED = '\033[41m'#(背景)赤 BG_GREEN = '\033[42m'#(背景)緑 BG_YELLOW = '\033[43m'#(背景)黄 BG_BLUE = '\033[44m'#(背景)青 BG_MAGENTA = '\033[45m'#(背景)マゼンタ BG_CYAN = '\033[46m'#(背景)シアン BG_WHITE = '\033[47m'#(背景)白 BG_DEFAULT = '\033[49m'#背景色をデフォルトに戻す RESET = '\033[0m'#全てリセット class Human(): """ 基底クラス """ name = "Human" attribute = "Human" tColor = Color.WHITE ; rColor = Color.RESET cWalk = 20; sWalk = "." def __init__(self, n ): self.name = n def Walk(self): s = "" for i in range(self.cWalk): s += self.sWalk print(self.attribute,"\t","w", self.tColor,s,"(",self.name,")",self.rColor) class Wizard(Human): name = "wizard" attribute = "Wizard" tColor = Color.MAGENTA ; rColor = Color.RESET cAttack = 20 ; sAttack = "@" cDifence = 10 ; sDifence = ":" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cDifence): s += self.sDifence print(self.attribute,"\t","D", self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cAttack): s += self.sAttack print(self.attribute,"\t", "A",self.tColor, s, "(" , self.name, ")", self.rColor ) class Shielder(Human): name = "Shield" attribute = "Shield" tColor = Color.GREEN ; rColor = Color.RESET cAttack = 20 ; sAttack = "→" cDifence = 10 ; sDifence = "-" def __init__(self, n ): self.name = n def Defense(self): s = "" for i in range(self.cDifence ): s += self.sDifence print(self.attribute,"\t","D", self.tColor, s, "(" , self.name, ")", self.rColor ) def Attack(self): s = "" for i in range(self.cAttack): s += self.sAttack print(self.attribute,"\t","A",self.tColor, s, "(" , self.name, ")", self.rColor ) class Swords(Human): name = "Swords" attribute = "Swords" tColor = Color.CYAN ; rColor = Color.RESET cAttack = 20 ; sAttack = "/" cDifence = 10 ; sDifence = ">" def __init__(self, n ): self.name = n def Defense(self): pass def Attack(self): pass class iAdventurer(metaclass=abc.ABCMeta): """ インターフェイス """ def Attack(self): pass def Defense(self): pass class subMain: #humanList = ["Nao*","Itsu*","Motoya","Re*"] """ インターフエイスの規定クラスとして登録 """ iAdventurer.register(Shielder) iAdventurer.register(Wizard) iAdventurer.register(Swords) # クラスをリスト化 Adventurers = [Shielder("Nao") , Wizard("Itsu"), Swords("Motoya")] for Adventurer in Adventurers: if isinstance(Adventurer, iAdventurer): print( f"属性:{Adventurer.attribute} 名前:{Adventurer.name} ") Adventurer.Attack() Adventurer.Defense() Adventurer.Walk() if __name__ == "__main__": root = subMain()